Generally we will validate the file types and file size of FileUpload Control at server side in ASP.NET.
The problem with this is, if you are uploading heavy file it will first load into the server memory through the browser and then checks the conditions at server side. To eliminate this we can check FileUpload control file type and file size at client side. Here I created one ASP.NET page how to restrict the file based on the file type and file size at client side.
1. Open file in browser.
2. Select invalid file which is not pdf/txt/doc/docx/excel file type.
3. Select the valid file size is more than 10MB.
4.Select the valid file and size is less than 10MB.
Page - UploadFile.aspx
<%@ Page Language="C#" AutoEventWireup="true" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <%--Refer the jQuery library into your web page--%> <script src="//ajax.googleapis.com/ajax/libs/jquery/1.10.2/jquery.min.js"></script> <script type="text/javascript"> $(document).ready(function () { /* Attach a change event to the file upload control to restrict the file type and file size on file selection. */ $("#fileupload").change(function () { // Get the file upload control file extension var ext = $(this).val().split('.').pop().toLowerCase(); // Create array with the files extensions to upload var fileListToUpload = new Array('pdf', 'txt', 'doc', 'docx', 'xls','xlsx'); //Check the file extension is in the array. var isValidFile = $.inArray(ext, fileListToUpload); // isValidFile gets the value -1 if the file extension is not in the list. if (isValidFile == -1) { ShowMessage('Please select a valid file of type pdf/txt/doc/docx/xls/xlsx.', 'error'); $(this).replaceWith($(this).val('').clone(true)); } else { // Restrict the file size to 10 MB. if ($(this).get(0).files[0].size > (1024 * 1024 * 10)) { ShowMessage('File size should not exceed 10MB.', 'error'); $(this).replaceWith($(this).val('').clone(true)); } else { ShowMessage('Thank you for selecting a valid file.', 'success'); } } }); // Dynamic function to show the messages on the page function ShowMessage(message, type) { if (type == 'success') { $("#fileStatus").removeClass('error'); $("#fileStatus").addClass('success'); } else if (type == 'error') { $("#fileStatus").removeClass('success'); $("#fileStatus").addClass('error'); } $("#fileStatus").text(message); } }); </script> <style> .error { color: red; font-size: 17px; } .success { color: green; font-size: 17px; } </style> </head> <body> <form id="formFile" runat="server"> <b>Upload File:</b> <asp:FileUpload ID="fileupload" runat="server" /><br /> <asp:Label ID="fileStatus" Text="" CssClass="error" runat="server" /> </form> </body> </html>
Test cases
1. Open file in browser.

2. Select invalid file which is not pdf/txt/doc/docx/excel file type.

3. Select the valid file size is more than 10MB.
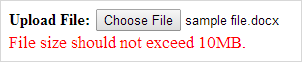
4.Select the valid file and size is less than 10MB.
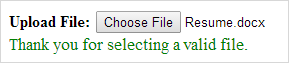